Table Of Content

In software engineering, the singleton pattern is a design pattern that is used to restrict instantiation of a class to one object. This is useful when exactly one object is needed to coordinate actions across the system. The concept is sometimes generalized to systems that operate more efficiently when only one object exists, or that restrict the instantiation to a certain number of objects (say, five). Some consider it an anti-pattern, judging that it is overused, introduces unnecessary limitations in situations where a sole instance of a class is not actually required, and introduces global state into an application. So, both threads will enter into the if condition and create new instances.
Singleton class vs. Static methods
Though there already are many posts available on Singleton design pattern, I'll try to cover this topic in the most simplistic way and easy to understand. The article will also talk about Static classes and the differences between singleton design pattern and static classes. In the above example, the PrintSpooler class ensures that only one instance is created using the concept of lazy initialization. The GetPrintSpooler method utilizes a lock to guarantee thread safety, ensuring that only one instance is created even in multi-threaded environments. In software engineering, the singleton pattern is a software design pattern that restricts the instantiation of a class to a singular instance.
What is the purpose of singleton pattern?

Now, let's test the above VoteMachine class in the multi-threaded scenario, as shown below. The following demonstrates the testing of the VoteMachine class in the multi-threaded environment using the Parallel class. Clients may not even realize that they’re working with the same object all the time. In this article, learn what a Singleton Design Pattern is and how to implement a Singleton Pattern in C#.
Singleton Class with Lazy Instantiation
The above singleton class uses the static property to return an instance of the class. It has a private parameterless constructor which will restrict the creation of an object using the new keyword. You can make the constructor protected if you want to allow it to be inherited in a subclass. The purpose of the singleton design pattern is to ensure that a class only has one instance and provide a global point of access to it throughout the life of an application. Access of one instance is preferred to avoid unexpected results.
Singleton Design Pattern C++ Example
After getting the design patterns, It is very easy to implement and build project architecture in real-time scenarios. The design patterns make the applications Reliable, Scalable, and easily Maintainable. Implementations of the singleton pattern ensure that only one instance of the singleton class ever exists and typically provide global access to that instance. Although there can be many printers in a system, there should be only one printer spooler. How do we ensure that a class has only one instance and that the instance is easily accessible? A global variable makes an object accessible, but it doesn’t keep you from instantiating multiple objects.
Logger class final result
First of all, we have to make our class constructor private so that we can not create objects from outside of the class using the new keyword. In this article, I will discuss the Singleton Design Pattern in C# with Examples. Please read our previous article discussing Shallow Copy and Deep Copy in C# with Examples. The Singleton Design Pattern in C# falls under the Creational Pattern Category.
The class can ensure that no other instance can be created (by intercepting requests to create new objects), and it can provide a way to access the instance. We have declared the obj volatile which ensures that multiple threads offer the obj variable correctly when it is being initialized to the Singleton instance. This method drastically reduces the overhead of calling the synchronized method every time.
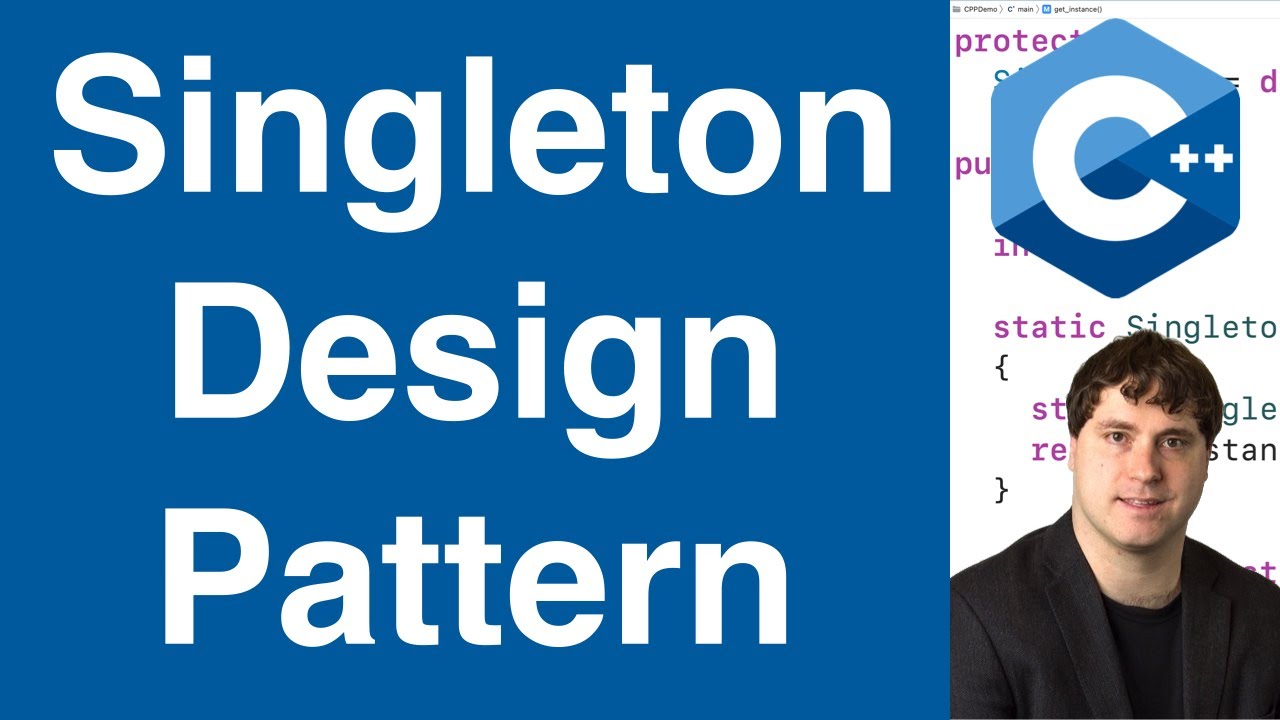
The right way to implement Singleton is by dependency injection, So instead of directly depending on a singleton, you might want to consider it depending on an abstraction(e.g. an interface). I would also encourage you to use synchronization primitives(like a mutex, semaphores, etc) to control access. You must know that Singleton instance doesn't need to be manually deleted by us. We need a single object of it throughout the whole program, so at the end of program execution, it will be automatically deallocated.
85 Must-Know C# Interview Questions and Answers [2024] - Simplilearn
85 Must-Know C# Interview Questions and Answers .
Posted: Mon, 15 Apr 2024 07:00:00 GMT [source]
The Logger class declaration
Thus I have a full control over the order of singletons creation/destruction, and also I guarantee that singletons will be created, no matter whether someone called getInstance() or not. [tl;dr] Modern C++ best practices allows an explicit and beautiful implementation of the singleton design pattern. In simple terms design patterns are practical solutions to general problems that software engineers faced during software development.
Top 30 C# OOPS Interview Questions and Answers for 2024 - Simplilearn
Top 30 C# OOPS Interview Questions and Answers for 2024.
Posted: Mon, 26 Sep 2022 08:07:50 GMT [source]
It relies on a little helper to enforce a single object at any one time (at most). To enforce a single object over program execution, remove the reset (which we find useful for tests). From this declaration, I can deduce that the instance field is initiated on the heap. What is completely unclear for me is when exactly the memory is going to be deallocated?
In one of my implementations, I called queue_ref() in AppDelegate.m (main.c would work, too). That way, queue will be initialized before any other calling object attempts to access it. Returning a value from a variable is much faster than calling a function, and then checking the value of the variable before returning it. I provide an explicit init() and term() methods for all the singletons and call them inside main.
And also, we can’t inherit this class into another class because of a private constructor. In this pattern, we have to restrict creating the instance if it is already created and use the existing one. The VoteMachine singleton class will work perfectly in the synchronous calls where each user will register their vote one by one. The following is the basic structure of the singleton class in C#. In the next article, I will discuss Why we Need to use the Sealed Keyword in the Singleton Class, as we already have a private constructor. In this article, I explain the basic concepts of the Singleton Design Pattern in C# with Examples.
Note that the performance degrades as a lock is acquired every time the instance is requested. Use thread lock before creating an object of a singleton class to make it thread-safe. The following checks whether the above Singleton class returns a single instance every time. This article will discuss the No Thread-Safe Singleton Design Pattern implementation in C#.
No comments:
Post a Comment